Image Gallery Slider
Example :
<!DOCTYPE html>
<html lang="en">
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Gallery</title>
<style>
.gallary-img{
width: 300px;
height: 200px;
object-fit: cover;
background-color: tomato;
display: block;
margin: 20px auto;
border-radius: 10px;
}
.btn-container{
position: relative;
width: fit-content;
display: block;
margin: 10px auto;
}
.btn{
height: 30px;
margin: 0 10px;
cursor: pointer;
background-color: #fff;
border-radius: 50%;
position: absolute;
top: 80px;
}
.btn-left{
left: -30px;
}
.btn-right{
right: -30px;
}
</style>
</head>
<body>
<div class="btn-container">
<img src="./gallery/one.jpg" class="gallary-img" id="img"/>
<img src="left-arrow.png" class="btn btn-left" onclick="prev()" />
<img src="arrow-right.png" class="btn btn-right" onclick="next()" />
</div>
<script>
const imagesArr = [
"./gallery/zero.jpg",
"./gallery/one.jpg",
"./gallery/two.jpg",
"./gallery/three.jpg",
"./gallery/four.jpg"
];
let imgIndex = 0;
const imgElement = document.getElementById('img');
function prev(){
if (imgIndex == 0) {
imgIndex = imagesArr.length - 1;
} else {
imgIndex--;
}
imgElement.src = imagesArr[imgIndex];
}
function next(){
if (imgIndex == imagesArr.length - 1) {
imgIndex = 0;
} else {
imgIndex++;
}
imgElement.src = imagesArr[imgIndex];
}
</script>
</body>
</html>
Output :
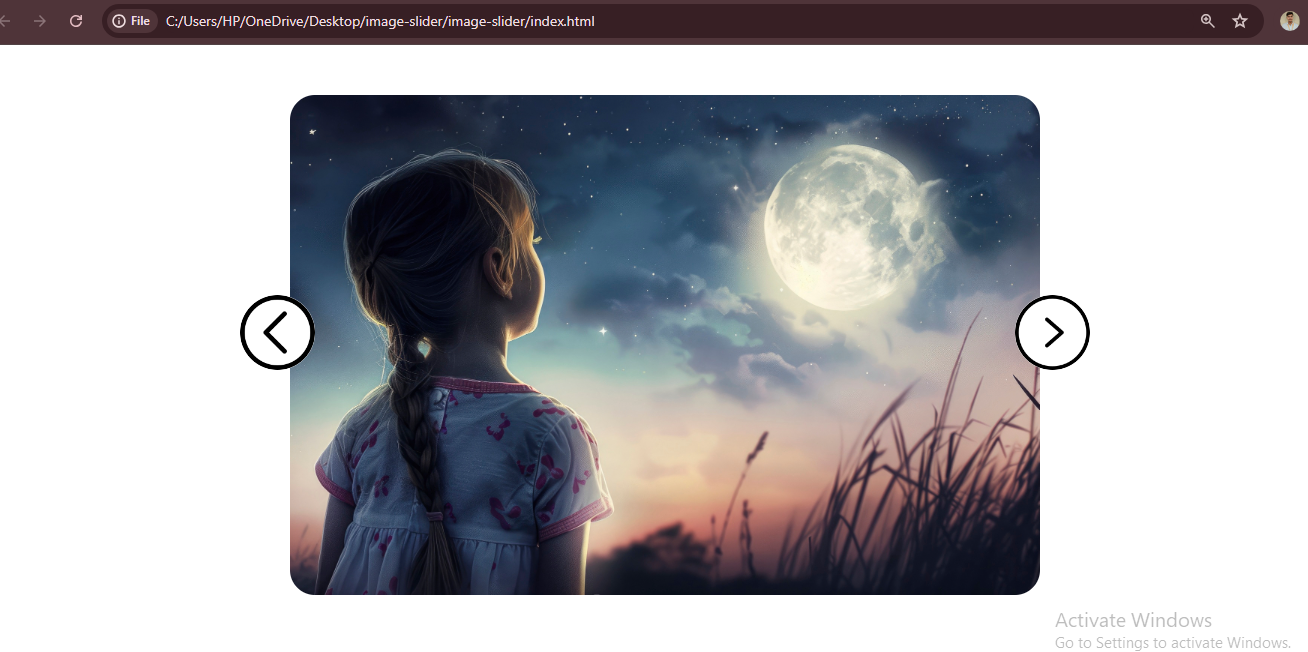
After click on next button or sign another image will display.
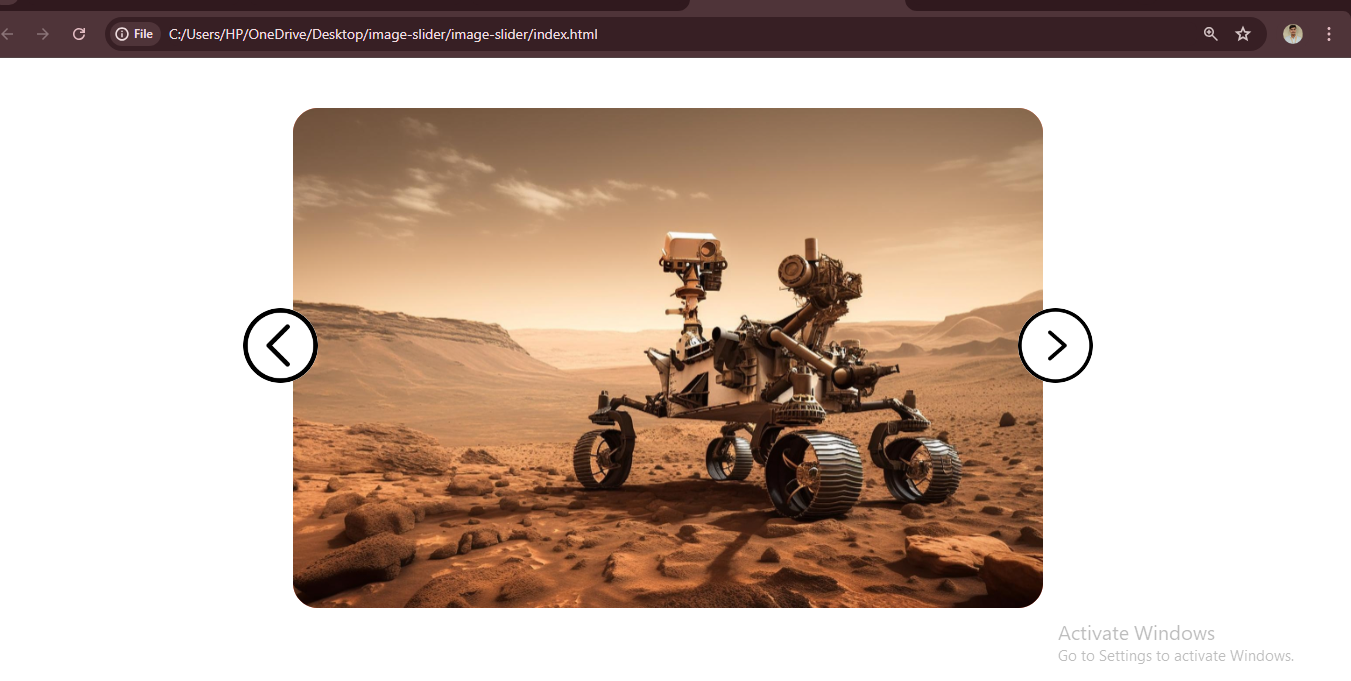
Explanation :
In the above example ,
const imagesArr = [ ... ];
This array containing the paths to all the images in the gallery.let imgIndex = 0;
It Initializes the index to keep track of the currently displayed image.const imgElement = document.getElementById('img');
Fetches the<img>
element with theid "img"
and stores it in theimgElement
constant.function prev() { ... }
Defines theprev()
function to show the previous image.
if (imgIndex == 0) { ... }
It Checks if the currentimgIndex
is0
.
imgIndex = imagesArr.length - 1;
If true, setsimgIndex
to the last image in the array.
else { imgIndex--; }
Otherwise, decrementsimgIndex
by 1.
imgElement.src = imagesArr[imgIndex];
It Updates thesrc
attribute ofimgElement
to display the previous image.function next() { ... }
It Defines thenext()
function to show the next image.
if (imgIndex == imagesArr.length - 1) { ... }
It Checks if the currentimgIndex
is the last image in the array.
imgIndex = 0;
If true, setsimgIndex
to0
.
else { imgIndex++; }
Otherwise, incrementsimgIndex
by1
.
imgElement.src = imagesArr[imgIndex];
It Updates thesrc
attribute ofimgElement
to display the next image.
Example without if else :
<!DOCTYPE html>
<html lang="en">
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Gallery</title>
<style>
.gallary-img{
width: 300px;
height: 200px;
object-fit: cover;
background-color: tomato;
display: block;
margin: 20px auto;
border-radius: 10px;
}
.btn-container{
position: relative;
width: fit-content;
display: block;
margin: 10px auto;
}
.btn{
height: 30px;
margin: 0 10px;
cursor: pointer;
background-color: #fff;
border-radius: 50%;
position: absolute;
top: 80px;
}
.btn-left{
left: -30px;
}
.btn-right{
right: -30px;
}
</style>
</head>
<body>
<div class="btn-container">
<img src="./gallery/one.jpg" class="gallary-img" id="img"/>
<img src="left-arrow.png" class="btn btn-left" onclick="prev()" />
<img src="arrow-right.png" class="btn btn-right" onclick="next()" />
</div>
<script>
const imagesArr = [
"./gallery/zero.jpg",
"./gallery/one.jpg",
"./gallery/two.jpg",
"./gallery/three.jpg",
"./gallery/four.jpg"
];
let imgIndex = 0;
const imgElement = document.getElementById('img');
function prev(){
imgIndex==0 ? imgIndex = imagesArr.length - 1 : imgIndex--;
imgElement.src=imagesArr[imgIndex]
}
function next(){
(imgIndex == imagesArr.length - 1) ? imgIndex = 0 : imgIndex++;
imgElement.src=imagesArr[imgIndex]
}
</script>
</body>
</html>
Explanation :
const imagesArr = [ ... ];
This array containing the paths to all the images in the gallery.let imgIndex = 0;
It Initializes the index to keep track of the currently displayed image.const imgElement = document.getElementById('img');
It Fetches the<img>
element with the id"img"
and stores it in theimgElement
constant.function prev(){ ... }
It Defines theprev()
function to show the previous image.imgIndex==0 ? imgIndex = imagesArr.length - 1 : imgIndex--;
It Checks if the currentimgIndex
is0
. If true, setsimgIndex
to the last image in the array. Otherwise, decrementsimgIndex
by1
imgElement.src=imagesArr[imgIndex];
It Updates thesrc
attribute ofimgElement
to display the previous image.function next(){ ... }
It Defines thenext()
function to show the next image.(imgIndex == imagesArr.length - 1) ? imgIndex = 0 : imgIndex++;
It Checks if the currentimgIndex
is the last image in the array. If true, setsimgIndex
to0
. Otherwise, incrementsimgIndex
by 1.imgElement.src=imagesArr[imgIndex];
It Updates thesrc
attribute ofimgElement
to display the next image.